Best Practices for Secure Programming in C++
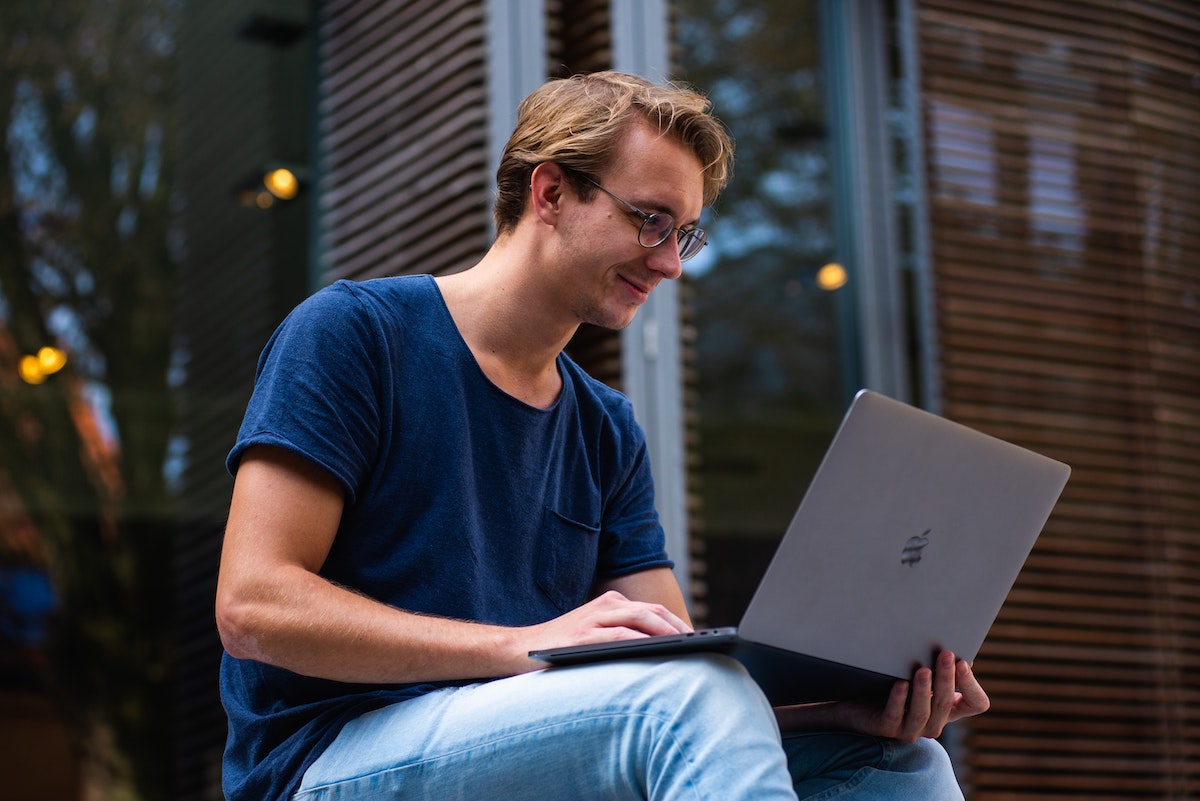
Despite the introduction of multiple programming languages over the past few years, C++ still remains one of the most powerful and widely used programming languages among developers. It’s widely known for its efficiency and performance, which allows developers to create reliable and high-performing applications.
However, like any other programming language, C++ faces security vulnerabilities. As a developer, secure programming should be among your top priorities during development. Secure programming ensures that you follow all the best practices available to maintain the integrity of the applications you’re developing. Whether you are developing small utility applications or working on complex systems, ensuring the security of your code is really important, as this will help protect user data while preventing issues like unauthorized access.
Common Security Threats in C++ Applications
As a C++ developer, being aware of the possible security threats you are bound to encounter will come in handy. With the correct understanding of the potential threats, you can easily address them while cleaning your code and ensuring its efficiency. Some of the most common threats include:
- Buffer overflow: This is the most common security concern associated with C++ applications. It occurs when a program writes data beyond the set bound of a buffer. This leads to the adjacent memory becoming corrupt. This threat can be exploited, leading to arbitrary code, overwriting critical data, or crashing the application.
- Integer overflow and underflow: This mostly occurs when the value you’re trying to store in an integer exceeds the maximum value that can be represented. Underflow occurs when the value is less than the minimum value that can be represented. This may lead to unexpected memory behavior, including corruption and potential breach. This includes use-after-free, double-free, and uninitialized memory access, which may lead to the exploitation of the program (by injecting malicious code or gaining unauthorized access).
- Injection attacks: This involves the insertion of malicious code into a program, which may lead to unintended execution. Some common types of injection associated with C++ include code injection and command injection.
- Pointer initialization: Using a pointer that is not correctly initialized may lead to the exposure of a lot of sensitive data. Additionally, if the uninitialized pointer is used, it may lead to the program reading or writing to an unexpected memory location.
- Incorrect type conversion: This is also known as type punning. It occurs when a program treats one data type as if it were a different one. This may cause data to be lost.
{{code-cta}}
Best Practices for Secure Coding in C++
Following best practices when developing applications using C++ will help you minimize vulnerabilities and also protect your applications against security threats.
- Input validation and sanitization: It’s always a good practice to validate and sanitize the user input. This will help prevent attacks such as SQL injection and command injections. Validation techniques such as regular expressions, input length checks, and input format validation can be used to enforce the expected input format. Additionally, make sure that you always validate and sanitize input from external sources.
- Memory management: Make use of memory management techniques such as dynamic memory allocation to prevent memory leaks and corruption. You should also consider utilizing smart pointers and “Resource Acquisition Is Initialization” (RAII) to automatically manage memory and resources, which will reduce the risks related to memory.
- Secure coding techniques: Be sure that you always use the secure standard libraries, functions, and containers such as std::vector, std::string, and std::unordered_map when writing code in C++. This helps handle memory management as well as prevent issues like buffer overflows and memory corruption.
- Error handling and logging: Implement proper error handling mechanisms to prevent information leakage in case of failures. Be sure to follow secure logging practices, which will help you maintain an audit trail and facilitate incident response.
- Access control and privileges: By enforcing proper access control, you will be able to limit user privileges and prevent unauthorized access to sensitive data by restricting permissions based on user roles and responsibilities. This can be achieved using techniques such as role-based access control (RBAC) or discretionary access control (DAC).
Examples of Secure Coding Techniques in C++
The techniques below will help you enhance the security of your C++ applications and thereby mitigate some common security threats.
Filter and Sanitize User Input
To protect your application from potential attacks, it’s a good practice to always validate user input. Below is an example of validating and filtering user input in which we validate the username against a set of allowed characters and lengths.
Use Smart Pointers and RAII
Smart pointers provide automatic memory management and help prevent memory leaks. Meanwhile, the RAII ensures timely resource deallocation. Below, you’ll see an example of acquiring and releasing resources along with automatic deallocation when it goes out of scope to prevent memory leaks.
Effective Error Handling
Proper error handling prevents information leakage. In the example below, the function throws an exception when the input value is negative. The exception is then handled in the main function, thus avoiding potential vulnerabilities.
Additional Considerations for Secure C++ Programming
In addition to best practices and secure coding techniques, there are still several considerations that C++ developers can implement to further enhance their application’s security. These include:
- Avoid using unsecured network protocols
- Implement secure authentication and authorization methods
- Avoid hardcoded secrets and sensitive info
- Regularly update and patch software dependencies
Conclusion
It’s important to note that secure programming is an ongoing process. Developers should stay up to date with the latest security practices and regularly update their code and libraries to address any known threats. By prioritizing security in the development process, developers can strengthen the security of their applications.
Investing time and effort in secure programming will not only help protect user data but also help strengthen trust among users and maintain the integrity of the system. Remember that writing secure C++ code should be your responsibility as a developer.
Add Mayhem to Your DevSecOps for Free.
Get a full-featured 30 day free trial.
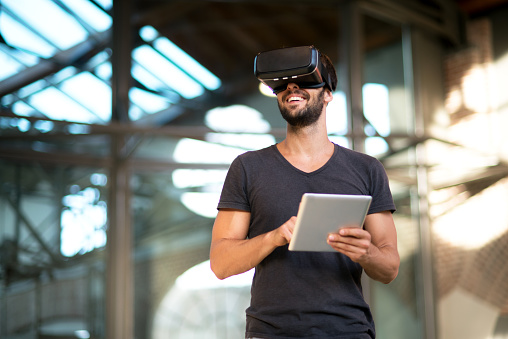